react-native-turboxml: Under the Hood of an Android-Native XML Parser
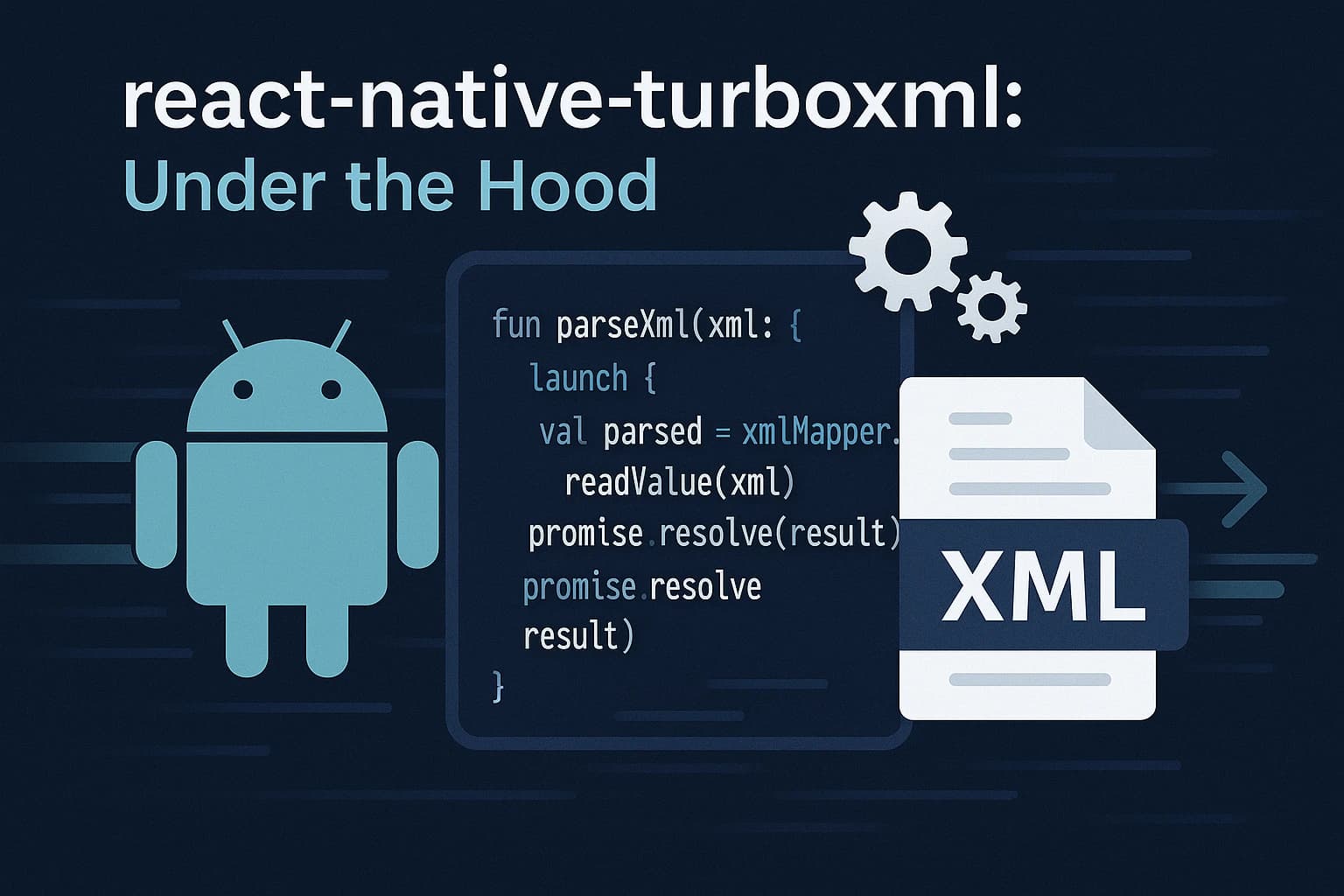
Introduction
Parsing multi-megabyte XML in JavaScript can block the React Native bridge and slow your app. react-native-turboxml moves that work into an Android-native TurboModule written in Kotlin. In this deep dive, you’ll see exactly how it works and why it’s so much faster.
1. Module Structure & Spec
The module implements the generated NativeTurboxmlSpec
interface. Its name "Turboxml"
makes it available to JavaScript as:
import { parseXml } from 'react-native-turboxml'
On Android, no manual C++ wiring is needed—React Native’s New Architecture toolchain generates the bridge.
2. parseXml
Implementation
The parseXml(xml: String): Promise<WritableMap>
method:
- Launches a coroutine on
Dispatchers.Default
(background thread) - Reads raw XML into a Kotlin
Map
via Jackson’sXmlMapper
- Cleans and normalizes the data
- Wraps it under the root tag and converts to React Native’s
WritableMap
- Resolves—or rejects—back on the main thread
override fun parseXml(xml: String, promise: Promise) { CoroutineScope(Dispatchers.Default).launch { try { val parsed = xmlMapper.readValue<Map<String, Any?>>(xml) val cleaned = clean(parsed) val normalized = normalize(cleaned) val rootTag = extractRoot(xml) val wrapped = mapOf(rootTag to normalized) val result = toWritableMap(wrapped) withContext(Dispatchers.Main) { promise.resolve(result) } } catch (e: Exception) { withContext(Dispatchers.Main) { promise.reject("XML_PARSE_ERROR", e.message, e) } } } }
3. Helper Functions
extractRoot
Uses a simple regex to find the top-level tag name.clean
Recursively removes blank keys, empty strings, and empty lists.normalize
Ensures every value is wrapped in a list or map for consistent output.toWritableMap
/toWritableArray
Convert Kotlin collections intoWritableMap
andWritableArray
for the JS bridge.
4. Multithreading on Android
By using Dispatchers.Default
, parsing runs on a background thread pool:
- Offloads heavy XML work away from the UI thread
- Makes use of all available CPU cores
- Keeps your app responsive even with very large inputs
5. Integration & Usage
-
Install
npm install react-native-turboxml # or yarn add react-native-turboxml
-
Enable New Architecture in React Native 0.71+ (Android only)
-
Import and use in your JS code:
import { parseXml } from 'react-native-turboxml' parseXml(xmlString) .then((data) => console.log(data)) .catch((err) => console.error('Parse error', err))
-
Avoid holding giant strings in JS if possible—consider splitting or streaming large inputs.
Conclusion
By offloading XML parsing into a Kotlin TurboModule, react-native-turboxml delivers:
- Non-blocking, multithreaded performance on Android
- Clean, JSON-like output via React Native maps and arrays
- Easy integration with React Native’s New Architecture
👉 Get started today:
npm install react-native-turboxml
Learn more at michaelouroumis.com/react-native-turboxml.